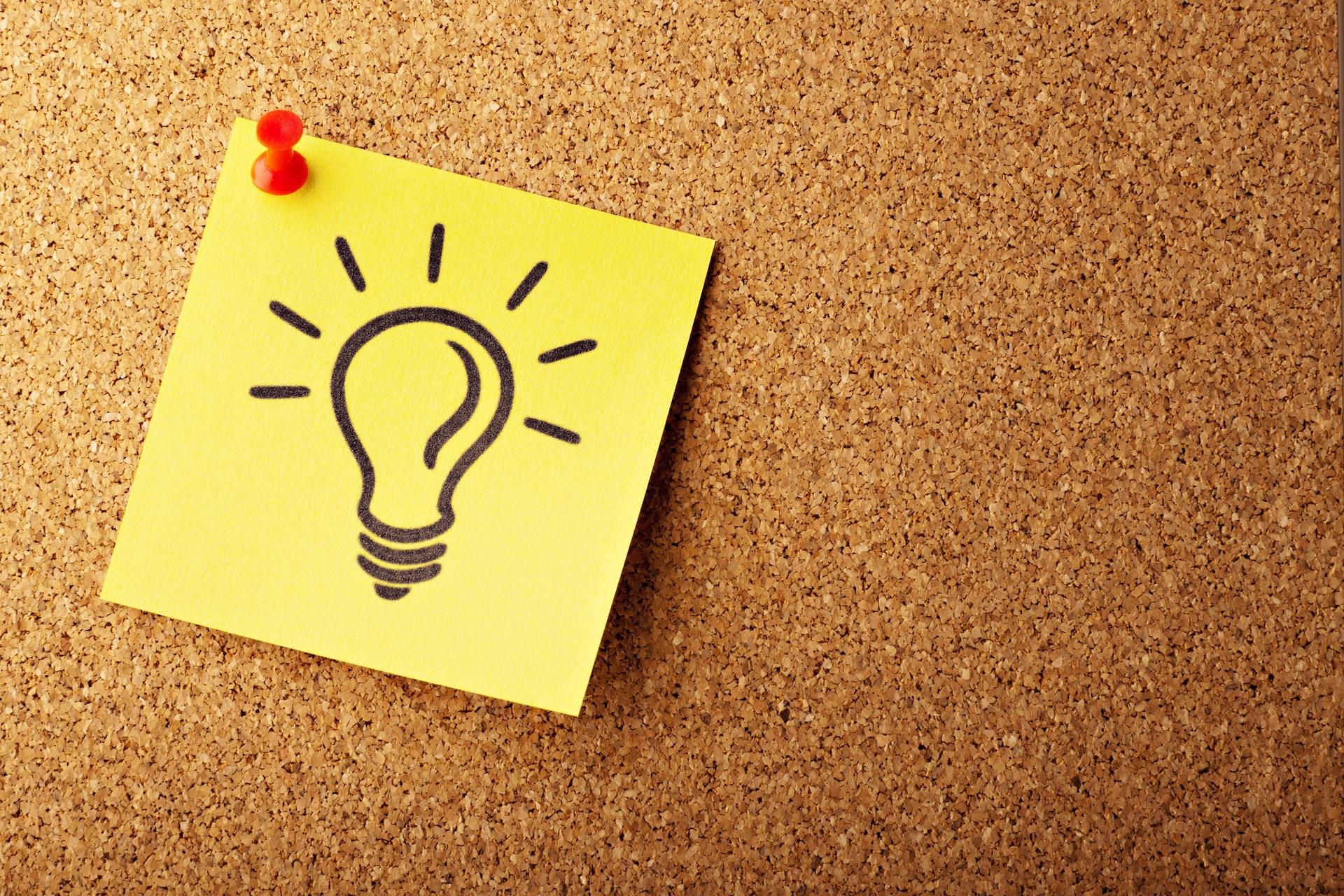
Some really good parts about JavaScript
Hold on Cowboy
This blog post is pretty old. Be careful with the information you find in here. The Times They Are A-Changin'
Most people who only know some jQuery and little JavaScript in the browser scratch their head and wonder how this lame language can be run on a server, or better yet, how most of your front end can be run on this messed up language.
JavaScript hits the nail on head
Functions as first class citizens.
So what does this mean? Functions can be embedded inside other functions
var outer = function embedded() {
var inside = function() {
var deep = 'variable inside a function, inside a function';
};
}
Notice how outer
has a function inside it called inside
, and inside the inside
function is a variable deep
? Did you see also how functions can be assigned to variables and even passed around.
I can even return a function from a function like this
function getMeSomeFunction() {
return function() {
alert('hey dude');
};
}
var hello = getMeSomeFunction();
hello() /// <---- this will alert 'hey dude'
Functions can be self executing like this
function() {
alert('now man');
}();
/// Notice the () at the end of the function... when JavaScript parses this, it will run it too.
Hoisting is pretty awesome too… you can use a function in file even before it’s been declared because JavaScript hoists all functions to the top before going through the rest of the code.
Scoping in JavaScript is far out
JavaScript allows you to scope things pretty nice, so you can include variables and have access to values in parent functions. An example is in order.
var foo = 'bar';
function wrapper() {
other();
}
function other() {
alert(foo);
}
/// Notice that other() is hoisted so you can use it before it's been declared.
/// Notice that inside other, you have access to foo
/// Notice that inside wrapper you have access to other()
There are better examples of great scoping, but right now I’m at the end of my post… tata for now.